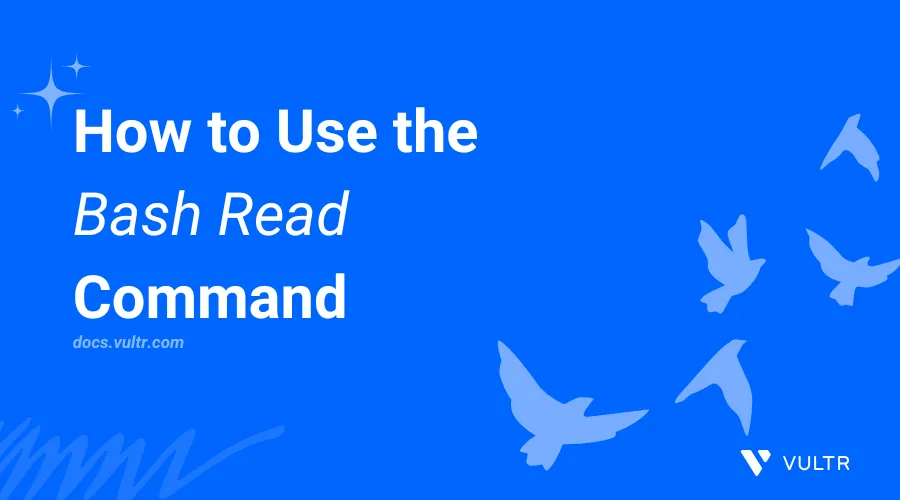
Introduction
The Bash read
command is a built-in command for capturing user input. The command reads text from the standard input and assigns the value to one or more variables. You can combine the bash read
command with conditional statements and loops to validate user input, read file contents, and perform specific tasks in a script.
This article explains how to use the Bash read
command. You'll use the command to create variables, read files, and validate user input in a shell or Bash script environment.
The Bash Read Command Syntax
The following is a basic read
command syntax in Bash.
read [options] [var_name]
In the above command:
[options]
: Includes optional flags that modify the command's behavior.var_name
: Includes the variable name that stores the input data.
The read
command supports the following options:
-p
: Displays a prompt before capturing the user input.-s
: Enables silent mode and hides input characters.-n
: Limits the number of input characters.-t
: Times out the input prompt after a specific number of seconds if the command receives no input.-a
: Stores the input into an array.-r
: Prevent backslashes (\
) from being interpreted by the shell as escape or special characters,
The read
command also supports multiple input values. You can store these values using separate variables with the following syntax.
read [options] [var_name] [var_name] [var_name]
To explore more on handling multiple conditions in Bash scripts, check out our guide on the bash case statement for effective pattern matching and control flow.
Capture User Input Using the Read Command
Follow the steps below to use the read
command to capture user input in a Bash shell environment.
Capture user input using the
What's your name
prompt and store the value in aname
variable.console$ read -p "What's your name:" name
Enter
John Doe
when prompted and press Enter to store the input in thename
variable.Print the variable using the
echo
command.console$ echo $name
Output:
John Doe
Run the following
read
command to store the user input in multiple variables, such asvar1
,var2
, andvar3
.console$ read -p "Enter the Input Values:" var1 var2 var3
Enter a string, such as
Hello World! This_Works
separated by spaces when prompted.Print the value of each variable.
console$ echo $var1 $var2 $var3
Output:
Hello World! This_Works
The bash
read
command assigns any extra values to the last variable when input values exceed the available variables. For example, with an input value such asHello World! This_works in bash
, the script assignsThis_works in bash
value to the lastvar3
variable.
Use the Bash Read Command with Redirections
The read
command works with STDIN (Standard Input) by default. You can redirect other commands' outputs to the read
command for processing and storage in a variable. This approach is important especially when working with files. Follow the steps below to use the read
command with redirections.
Create a new
greet
variable using theread
command and aHello World! Greetings from Vultr
string<<<
redirection.console$ read greet <<< "Hello World! Greetings from Vultr"
Print the
greet
variable.console$ echo $greet
Output:
Hello World! Greetings from Vultr
Create a new
greeting
variable and redirect its value to theread
command.console$ greeting="Hello World! Bash works!"; read greet <<< "$greeting"; echo $greet
Output:
Hello World! Bash works!
Create a new
readme.txt
file to use with theread
command.console$ nano readme.txt
Add the following contents to the
readme.txt
file.textHello World! Greetings from Vultr. This is a sample README script
Save and close the file.
Display the contents of the
readme.txt
file using theread
command and awhile
loop.console$ i=1; while read -r line; do echo "$i: $line"; ((i++)); done < readme.txt
The above
while
loop uses theread
command to display all lines in thereadme.txt
input file. The loop increments thei
variable using the((i++))
statement to number each line in the output.Output:
1: Hello World! 2: Greetings from Vultr. 3: This is a sample README script
Use IFS and the Read Command to Split Input
IFS (Internal Field Separator) is a built-in Bash variable that splits characters into separate strings or fields. IFS enables the read
command to split multiple inputs using spaces, tabs, and newlines delimiters by default. In the following steps, use IFS to enable other characters as delimiters to split user input with the read
command.
IFS uses the following inline syntax with the read
command unless you specify a delimiter in the global variable.
IFS='delimiter' read [options] [var_name]
Input a new string, such as
Hello World! This_works
separated by spaces using theread
command to split and store the values in multiple variables.console$ read var1 var2 var3 <<< "Hello World! This_works"
By default, IFS uses spaces as the default delimiter for the
read
command.Print the value of each variable.
console$ echo $var1 $var2 $var3
Output:
Hello World! This_works
Set a new IFS delimiter, such as
:
to modify theread
command's split method.console$ IFS=':'
Input the
Hello World! This_works
string again.console$ read var1 var2 var3 <<< "Hello World! This_works"
Print the value of the first variable. This time, the read command assigns the text to the first variable because you changed the delimiter.
console$ echo $var1
Output:
Hello World! This_works
Input the
Hello:World:This_works
string again and separate the string using the:
delimiter.console$ read var1 var2 var3 <<< "Hello:World:This_works"
Print the value of the first variable again.
console$ echo $var1
Output:
Hello
Print the value of each variable.
console$ echo $var1 $var2 $var3
Output:
Hello World This_works
Use IFS and the
read
command with multiple delimiters such as:
,_
, and-
to split the input.console$ echo 'Hello:World-This_works.' | (IFS=":-_-" read -r var1 var2 var3; echo -e "$var1 $var2 $var3")
Output:
Hello World This_works.
Reset IFS to the default delimiter settings.
console$ IFS=$' \t\n'
Use the Read Command with Arrays in Bash
The bash read
command stores multiple values into arrays using the -a
option and splits the input using the default delimiter. Follow the steps below to use the read
command with arrays in Bash.
Input a new
Hello World! This Works
string value and store the input in anew_array
array.console$ read -a new_array <<< "Hello World! This Works"
Print all elements in the array.
console$ echo "${new_array[@]}"
Output:
Hello World! This Works
Create a new
del_array
array and set:
as the delimiter using IFS to split the input characters.console$ input="Hello:World:This:Works"; IFS=':' read -r -a del_array <<< "$input"
Print all elements in the array.
console$ echo "${del_array[@]}"
Output:
Hello World This Works
Validate User Input Using the Read Command and Conditional Statements in Bash
The read
command captures and assigns user inputs to variables. You can use these variables to perform specific tasks like validating user input using conditional statements by following the steps below.
Create a new
validate.sh
script.console$ nano validate.sh
Add the following contents to the
validate.sh
file.bash#!/bin/bash admins=("user1" "user2" "user3") read -p "Enter your username: " name if [[ " ${admins[@]} " =~ " $name " ]]; then echo "You have sudo user privileges!" else echo "Access denied, you don't have sudo user privileges" fi
Save and close the file.
The above script compares the user input from the
name
variable using theif-else
conditional statement. If the condition returnstrue
and the name matches any element in theadmins
array, the script executes the following commands.Run the script using Bash.
console$ bash validate.sh
Enter a valid user, such as
user1
when prompted.Enter your username:
Output:
You have sudo user privileges!
Conclusion
You have used the bash read
command to capture and validate user input. You have also combined the read
command with loops, conditional expressions, and arrays to perform specific tasks. The read
command is important when automating Bash scripts that require user input to perform specific actions on a system.
No comments yet.