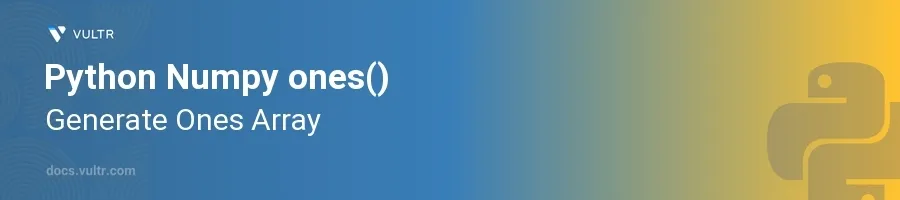
Introduction
In scientific computing and data analysis, generating arrays with default values is a common necessity. The numpy
library in Python offers various functions to efficiently create and manipulate large arrays, with ones()
being one of its basic yet powerful tools. This function generates arrays filled entirely with the value 1, which is particularly handy in matrix operations and other algebraic computations where identity matrices or neutral elements are required.
In this article, you will learn how to use the numpy
module's ones()
function to create arrays filled with the number 1. Key examples will demonstrate how to customize these arrays in terms of shape, data type, and specific use cases, such as setting up matrices for linear algebra operations.
Creating Basic Ones Arrays
Generate a Simple One-Dimensional Array
Import the
numpy
module.Use the
ones()
function to create an array.pythonimport numpy as np one_d_array = np.ones(5) print(one_d_array)
This code creates a one-dimensional array of length 5, with all elements set to 1.
Customizing the Data Type
Specify the data type using the
dtype
parameter.pythonone_d_float_array = np.ones(5, dtype=float) print(one_d_float_array)
By default,
np.ones()
creates arrays of data typefloat64
. Here, you specifyfloat
explicitly, ensuring the array elements are floating-point numbers.
Generating Multi-Dimensional Arrays
Creating a Two-Dimensional Array
Define the shape of the array as a tuple.
Generate the two-dimensional array using the shape.
pythontwo_d_array = np.ones((3, 4)) print(two_d_array)
This snippet constructs a 3x4 matrix, with all elements initialized to 1.
Using ones()
in Higher Dimensional Arrays
Specify a tuple with more dimensions for the shape.
pythonthree_d_array = np.ones((2, 3, 4)) print(three_d_array)
This code illustrates how to generate a three-dimensional array filled with 1s, with dimensions specified as 2x3x4.
Practical Applications of the ones()
Function
Setting Up an Identity Matrix
Understand that identity matrices are square matrices with 1s on the diagonal and 0s elsewhere.
Use
ones()
withnp.eye()
to generate an identity matrix.pythonn = 4 identity_matrix = np.eye(n) * np.ones((n, n)) print(identity_matrix)
This setup effectively multiplies a size
n
identity matrix by a matrix of ones of the same size, achieving an identity matrix usingones()
.
Allocating Memory for Algorithms
Utilize
ones()
to initialize arrays that are to be used in iterative algorithms.pythoninitial_values = np.ones(10) print("Initial values for algorithm:", initial_values)
Here, you generate an array filled with 1s to serve as initial values or conditions for iterative calculations in algorithms.
Conclusion
The ones()
function in numpy
is an essential utility for generating arrays filled with the value 1 across a variety of sizes and dimensions. From creating simple vectors and matrices to initializing data structures for complex computational algorithms, this function adds efficiency and simplicity to Python code. By mastering the use of ones()
, enhance the setup of mathematical models and data processing tasks, ensuring uniformity and correctness in computational operations.
No comments yet.