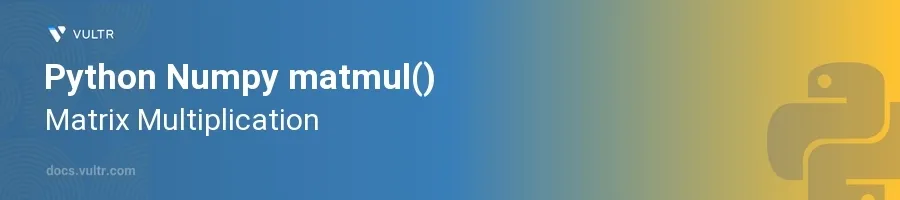
Introduction
The matmul()
function in the NumPy library is a critical tool for performing matrix multiplication, which is a foundational operation in linear algebra and is heavily utilized in fields such as data science, physics, and engineering. Matrix multiplication is not just a simple element-wise operation but involves a calculation between rows and columns of the matrices involved.
In this article, you will learn how to efficiently use the matmul()
function for matrix multiplication in Python. Discover the nuances of working with 2D arrays and higher dimensional data, ensuring that your matrix operations are performed correctly and efficiently.
Understanding Matrix Multiplication
Basic Concept of Matrix Multiplication
- Recognize that matrix multiplication involves the dot product of rows and columns.
- Understand the requirement for the inner dimensions of the matrices to match.
Using matmul() for 2D Arrays
Ensure both matrices have compatible dimensions.
Apply the
matmul()
function.pythonimport numpy as np matrix_a = np.array([[1, 2], [3, 4]]) matrix_b = np.array([[2, 0], [1, 2]]) result = np.matmul(matrix_a, matrix_b)
This code multiplies a 2x2 matrix
matrix_a
with another 2x2 matrixmatrix_b
. The resulting matrix will also be a 2x2 matrix representing the dot product of rows frommatrix_a
and columns frommatrix_b
.
Applying matmul() in Different Scenarios
Multiplying Higher Dimensional Arrays
Use
matmul()
when dealing with tensors (3D or higher).Integrate
matmul()
in tensor algebra to exploit its properties for complex calculations.pythontensor_a = np.random.rand(2, 2, 2) tensor_b = np.random.rand(2, 2, 2) result_tensor = np.matmul(tensor_a, tensor_b)
Here,
matmul()
computes the multiplication operation across the last two axes of each tensor, essential when dealing with multi-dimensional data in advanced computation like neural network operations.
Batch Matrix Multiplication
Perform matrix multiplication over batches of matrices using the same
matmul()
function.Utilize the advantage of
matmul()
when computing products of several matrix pairs in a batch.pythonbatch_a = np.random.rand(10, 2, 3) batch_b = np.random.rand(10, 3, 2) batch_result = np.matmul(batch_a, batch_b)
Each pair of matrices (from
batch_a
andbatch_b
) within the batch undergoes multiplication resulting in a new batch of resultant matrices, which is extremely useful for operations like batch processing in machine learning models.
Conclusion
The matmul()
function from NumPy is a versatile tool indispensable for anyone involved with linear algebra and numerical computations in Python. It supports not only two-dimensional matrix multiplication but also extends to higher-dimensional data, making it an excellent choice for a wide array of scientific and engineering applications. By mastering the matmul()
function, you ensure your computations are robust, efficient, and scalable. Whether dealing with basic matrix operations or complex multi-dimensional batch processes, matmul()
is crucial for handling linear transformations and other matrix-related calculations effectively.
No comments yet.